Gemini मॉडल, इमेज को प्रोसेस कर सकते हैं. इससे डेवलपर के कई ऐसे इस्तेमाल के उदाहरणों को पूरा किया जा सकता है जिनके लिए पहले डोमेन के हिसाब से मॉडल की ज़रूरत होती थी. Gemini की विज़न की कुछ सुविधाओं में ये शामिल हैं:
- इमेज के कैप्शन जोड़ना और उनसे जुड़े सवालों के जवाब देना
- PDF फ़ाइलों को टेक्स्ट में बदलना और उनमें मौजूद 20 लाख तक टोकन की वजह बताना
- किसी इमेज में मौजूद ऑब्जेक्ट का पता लगाना और उनके लिए बाउंडिंग बॉक्स के निर्देशांक दिखाना
- इमेज में मौजूद ऑब्जेक्ट को सेगमेंट में बांटना
Gemini को शुरुआत से ही मल्टीमोडल के तौर पर बनाया गया था. हम इसकी नई-नई संभावनाओं को तलाशते रहते हैं. इस गाइड में, इमेज इनपुट के आधार पर टेक्स्ट रिस्पॉन्स जनरेट करने और इमेज को समझने से जुड़े सामान्य टास्क करने के लिए, Gemini API का इस्तेमाल करने का तरीका बताया गया है.
शुरू करने से पहले
Gemini API को कॉल करने से पहले, पक्का करें कि आपने अपने पसंदीदा एसडीके टूल को इंस्टॉल कर लिया हो. साथ ही, Gemini API पासकोड को कॉन्फ़िगर कर लिया हो और वह इस्तेमाल के लिए तैयार हो.
इमेज इनपुट
Gemini को इनपुट के तौर पर इमेज देने के लिए, ये तरीके अपनाए जा सकते हैं:
generateContent
से अनुरोध करने से पहले, File API का इस्तेमाल करके इमेज फ़ाइल अपलोड करें. 20 एमबी से बड़ी फ़ाइलों के लिए या एक से ज़्यादा अनुरोधों में फ़ाइल का फिर से इस्तेमाल करने के लिए, यह तरीका अपनाएं.generateContent
के अनुरोध के साथ इनलाइन इमेज डेटा पास करें. इस तरीके का इस्तेमाल, छोटी फ़ाइलों या सीधे यूआरएल से फ़ेच की गई इमेज के लिए करें. फ़ाइलों का कुल अनुरोध साइज़ 20 एमबी से कम होना चाहिए.
इमेज फ़ाइल अपलोड करना
इमेज फ़ाइल अपलोड करने के लिए, Files API का इस्तेमाल किया जा सकता है. जब अनुरोध का कुल साइज़ (इसमें फ़ाइल, टेक्स्ट प्रॉम्प्ट, सिस्टम के निर्देश वगैरह शामिल हैं) 20 एमबी से ज़्यादा हो, तो Files API का हमेशा इस्तेमाल करें. इसके अलावा, अगर आपको एक ही इमेज का इस्तेमाल कई प्रॉम्प्ट में करना है, तो भी Files API का इस्तेमाल करें.
यह कोड, इमेज फ़ाइल अपलोड करता है. इसके बाद, generateContent
को कॉल करने के लिए फ़ाइल का इस्तेमाल करता है.
Python
from google import genai
client = genai.Client(api_key="GOOGLE_API_KEY")
myfile = client.files.upload(file="path/to/sample.jpg")
response = client.models.generate_content(
model="gemini-2.0-flash",
contents=[myfile, "Caption this image."])
print(response.text)
JavaScript
import {
GoogleGenAI,
createUserContent,
createPartFromUri,
} from "@google/genai";
const ai = new GoogleGenAI({ apiKey: "GOOGLE_API_KEY" });
async function main() {
const myfile = await ai.files.upload({
file: "path/to/sample.jpg",
config: { mimeType: "image/jpeg" },
});
const response = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: createUserContent([
createPartFromUri(myfile.uri, myfile.mimeType),
"Caption this image.",
]),
});
console.log(response.text);
}
await main();
शुरू करें
file, err := client.UploadFileFromPath(ctx, "path/to/sample.jpg", nil)
if err != nil {
log.Fatal(err)
}
defer client.DeleteFile(ctx, file.Name)
model := client.GenerativeModel("gemini-2.0-flash")
resp, err := model.GenerateContent(ctx,
genai.FileData{URI: file.URI},
genai.Text("Caption this image."))
if err != nil {
log.Fatal(err)
}
printResponse(resp)
REST
IMAGE_PATH="path/to/sample.jpg"
MIME_TYPE=$(file -b --mime-type "${IMAGE_PATH}")
NUM_BYTES=$(wc -c < "${IMAGE_PATH}")
DISPLAY_NAME=IMAGE
tmp_header_file=upload-header.tmp
# Initial resumable request defining metadata.
# The upload url is in the response headers dump them to a file.
curl "https://generativelanguage.googleapis.com/upload/v1beta/files?key=${GOOGLE_API_KEY}" \
-D upload-header.tmp \
-H "X-Goog-Upload-Protocol: resumable" \
-H "X-Goog-Upload-Command: start" \
-H "X-Goog-Upload-Header-Content-Length: ${NUM_BYTES}" \
-H "X-Goog-Upload-Header-Content-Type: ${MIME_TYPE}" \
-H "Content-Type: application/json" \
-d "{'file': {'display_name': '${DISPLAY_NAME}'}}" 2> /dev/null
upload_url=$(grep -i "x-goog-upload-url: " "${tmp_header_file}" | cut -d" " -f2 | tr -d "\r")
rm "${tmp_header_file}"
# Upload the actual bytes.
curl "${upload_url}" \
-H "Content-Length: ${NUM_BYTES}" \
-H "X-Goog-Upload-Offset: 0" \
-H "X-Goog-Upload-Command: upload, finalize" \
--data-binary "@${IMAGE_PATH}" 2> /dev/null > file_info.json
file_uri=$(jq ".file.uri" file_info.json)
echo file_uri=$file_uri
# Now generate content using that file
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{"file_data":{"mime_type": "${MIME_TYPE}", "file_uri": '$file_uri'}},
{"text": "Caption this image."}]
}]
}' 2> /dev/null > response.json
cat response.json
echo
jq ".candidates[].content.parts[].text" response.json
मीडिया फ़ाइलों के साथ काम करने के बारे में ज़्यादा जानने के लिए, Files API देखें.
इमेज का डेटा इनलाइन पास करना
इमेज फ़ाइल अपलोड करने के बजाय, generateContent
को अनुरोध में इनलाइन इमेज डेटा पास किया जा सकता है. यह छोटी इमेज (कुल अनुरोध का साइज़ 20 एमबी से कम) या सीधे यूआरएल से फ़ेच की गई इमेज के लिए सही है.
इमेज का डेटा, Base64 एन्कोड की गई स्ट्रिंग के तौर पर या सीधे तौर पर लोकल फ़ाइलें पढ़कर दिया जा सकता है. यह SDK टूल पर निर्भर करता है.
लोकल इमेज फ़ाइल:
Python
from google.genai import types
with open('path/to/small-sample.jpg', 'rb') as f:
img_bytes = f.read()
response = client.models.generate_content(
model='gemini-2.0-flash',
contents=[
types.Part.from_bytes(
data=img_bytes,
mime_type='image/jpeg',
),
'Caption this image.'
]
)
print(response.text)
JavaScript
import { GoogleGenAI } from "@google/genai";
import * as fs from "node:fs";
const ai = new GoogleGenAI({ apiKey: "GOOGLE_API_KEY" });
const base64ImageFile = fs.readFileSync("path/to/small-sample.jpg", {
encoding: "base64",
});
const contents = [
{
inlineData: {
mimeType: "image/jpeg",
data: base64ImageFile,
},
},
{ text: "Caption this image." },
];
const response = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: contents,
});
console.log(response.text);
शुरू करें
model := client.GenerativeModel("gemini-2.0-flash")
bytes, err := os.ReadFile("path/to/small-sample.jpg")
if err != nil {
log.Fatal(err)
}
prompt := []genai.Part{
genai.Blob{MIMEType: "image/jpeg", Data: bytes},
genai.Text("Caption this image."),
}
resp, err := model.GenerateContent(ctx, prompt...)
if err != nil {
log.Fatal(err)
}
for _, c := range resp.Candidates {
if c.Content != nil {
fmt.Println(*c.Content)
}
}
REST
IMG_PATH=/path/to/your/image1.jpg
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{
"inline_data": {
"mime_type":"image/jpeg",
"data": "'\$(base64 \$B64FLAGS \$IMG_PATH)'"
}
},
{"text": "Caption this image."},
]
}]
}' 2> /dev/null
यूआरएल से इमेज:
Python
from google import genai
from google.genai import types
import requests
image_path = "https://goo.gle/instrument-img"
image = requests.get(image_path)
client = genai.Client(api_key="GOOGLE_API_KEY")
response = client.models.generate_content(
model="gemini-2.0-flash-exp",
contents=["What is this image?",
types.Part.from_bytes(data=image.content, mime_type="image/jpeg")])
print(response.text)
JavaScript
import { GoogleGenAI } from "@google/genai";
async function main() {
const ai = new GoogleGenAI({ apiKey: process.env.GOOGLE_API_KEY });
const imageUrl = "https://goo.gle/instrument-img";
const response = await fetch(imageUrl);
const imageArrayBuffer = await response.arrayBuffer();
const base64ImageData = Buffer.from(imageArrayBuffer).toString('base64');
const result = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: [
{
inlineData: {
mimeType: 'image/jpeg',
data: base64ImageData,
},
},
{ text: "Caption this image." }
],
});
console.log(result.text);
}
main();
शुरू करें
func main() {
ctx := context.Background()
client, err := genai.NewClient(ctx, option.WithAPIKey(os.Getenv("GOOGLE_API_KEY")))
if err != nil {
log.Fatal(err)
}
defer client.Close()
model := client.GenerativeModel("gemini-2.0-flash")
// Download the image.
imageResp, err := http.Get("https://goo.gle/instrument-img")
if err != nil {
panic(err)
}
defer imageResp.Body.Close()
imageBytes, err := io.ReadAll(imageResp.Body)
if err != nil {
panic(err)
}
// Create the request.
req := []genai.Part{
genai.ImageData("jpeg", imageBytes),
genai.Text("Caption this image."),
}
// Generate content.
resp, err := model.GenerateContent(ctx, req...)
if err != nil {
panic(err)
}
// Handle the response of generated text.
for _, c := range resp.Candidates {
if c.Content != nil {
fmt.Println(*c.Content)
}
}
}
REST
IMG_URL="https://goo.gle/instrument-img"
MIME_TYPE=$(curl -sIL "$IMG_URL" | grep -i '^content-type:' | awk -F ': ' '{print $2}' | sed 's/\r$//' | head -n 1)
if [[ -z "$MIME_TYPE" || ! "$MIME_TYPE" == image/* ]]; then
MIME_TYPE="image/jpeg"
fi
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{
"inline_data": {
"mime_type":"'"$MIME_TYPE"'",
"data": "'$(curl -sL "$IMG_URL" | base64 $B64FLAGS)'"
}
},
{"text": "Caption this image."}
]
}]
}' 2> /dev/null
इनलाइन इमेज डेटा के बारे में ध्यान रखने वाली कुछ बातें:
- अनुरोध का कुल साइज़ 20 एमबी से ज़्यादा नहीं होना चाहिए. इसमें टेक्स्ट प्रॉम्प्ट, सिस्टम के निर्देश, और इनलाइन में दी गई सभी फ़ाइलें शामिल हैं. अगर आपकी फ़ाइल के साइज़ की वजह से, अनुरोध का कुल साइज़ 20 एमबी से ज़्यादा हो जाता है, तो अनुरोध में इस्तेमाल करने के लिए, Files API का इस्तेमाल करके इमेज फ़ाइल अपलोड करें.
- अगर किसी इमेज सैंपल का कई बार इस्तेमाल किया जा रहा है, तो File API का इस्तेमाल करके इमेज फ़ाइल अपलोड करना ज़्यादा बेहतर होता है.
एक से ज़्यादा इमेज के साथ प्रॉम्प्ट करना
contents
कलेक्शन में कई इमेज Part
ऑब्जेक्ट शामिल करके, एक ही प्रॉम्प्ट में कई इमेज दी जा सकती हैं. इनमें इनलाइन डेटा (लोकल फ़ाइलें या यूआरएल) और File API रेफ़रंस का इस्तेमाल किया जा सकता है.
Python
from google import genai
from google.genai import types
client = genai.Client(api_key="GOOGLE_API_KEY")
# Upload the first image
image1_path = "path/to/image1.jpg"
uploaded_file = client.files.upload(file=image1_path)
# Prepare the second image as inline data
image2_path = "path/to/image2.png"
with open(image2_path, 'rb') as f:
img2_bytes = f.read()
# Create the prompt with text and multiple images
response = client.models.generate_content(
model="gemini-2.0-flash",
contents=[
"What is different between these two images?",
uploaded_file, # Use the uploaded file reference
types.Part.from_bytes(
data=img2_bytes,
mime_type='image/png'
)
]
)
print(response.text)
JavaScript
import {
GoogleGenAI,
createUserContent,
createPartFromUri,
} from "@google/genai";
import * as fs from "node:fs";
const ai = new GoogleGenAI({ apiKey: "GOOGLE_API_KEY" });
async function main() {
// Upload the first image
const image1_path = "path/to/image1.jpg";
const uploadedFile = await ai.files.upload({
file: image1_path,
config: { mimeType: "image/jpeg" },
});
// Prepare the second image as inline data
const image2_path = "path/to/image2.png";
const base64Image2File = fs.readFileSync(image2_path, {
encoding: "base64",
});
// Create the prompt with text and multiple images
const response = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: createUserContent([
"What is different between these two images?",
createPartFromUri(uploadedFile.uri, uploadedFile.mimeType),
{
inlineData: {
mimeType: "image/png",
data: base64Image2File,
},
},
]),
});
console.log(response.text);
}
await main();
शुरू करें
+ // Upload the first image
image1Path := "path/to/image1.jpg"
uploadedFile, err := client.UploadFileFromPath(ctx, image1Path, nil)
if err != nil {
log.Fatal(err)
}
defer client.DeleteFile(ctx, uploadedFile.Name)
// Prepare the second image as inline data
image2Path := "path/to/image2.png"
img2Bytes, err := os.ReadFile(image2Path)
if err != nil {
log.Fatal(err)
}
// Create the prompt with text and multiple images
model := client.GenerativeModel("gemini-2.0-flash")
prompt := []genai.Part{
genai.Text("What is different between these two images?"),
genai.FileData{URI: uploadedFile.URI},
genai.Blob{MIMEType: "image/png", Data: img2Bytes},
}
resp, err := model.GenerateContent(ctx, prompt...)
if err != nil {
log.Fatal(err)
}
printResponse(resp)
REST
# Upload the first image
IMAGE1_PATH="path/to/image1.jpg"
MIME1_TYPE=$(file -b --mime-type "${IMAGE1_PATH}")
NUM1_BYTES=$(wc -c < "${IMAGE1_PATH}")
DISPLAY_NAME1=IMAGE1
tmp_header_file1=upload-header1.tmp
curl "https://generativelanguage.googleapis.com/upload/v1beta/files?key=${GOOGLE_API_KEY}" \
-D upload-header1.tmp \
-H "X-Goog-Upload-Protocol: resumable" \
-H "X-Goog-Upload-Command: start" \
-H "X-Goog-Upload-Header-Content-Length: ${NUM1_BYTES}" \
-H "X-Goog-Upload-Header-Content-Type: ${MIME1_TYPE}" \
-H "Content-Type: application/json" \
-d "{'file': {'display_name': '${DISPLAY_NAME1}'}}" 2> /dev/null
upload_url1=$(grep -i "x-goog-upload-url: " "${tmp_header_file1}" | cut -d" " -f2 | tr -d "\r")
rm "${tmp_header_file1}"
curl "${upload_url1}" \
-H "Content-Length: ${NUM1_BYTES}" \
-H "X-Goog-Upload-Offset: 0" \
-H "X-Goog-Upload-Command: upload, finalize" \
--data-binary "@${IMAGE1_PATH}" 2> /dev/null > file_info1.json
file1_uri=$(jq ".file.uri" file_info1.json)
echo file1_uri=$file1_uri
# Prepare the second image (inline)
IMAGE2_PATH="path/to/image2.png"
MIME2_TYPE=$(file -b --mime-type "${IMAGE2_PATH}")
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
IMAGE2_BASE64=$(base64 $B64FLAGS $IMAGE2_PATH)
# Now generate content using both images
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{"text": "What is different between these two images?"},
{"file_data":{"mime_type": "'"${MIME1_TYPE}"'", "file_uri": '$file1_uri'}},
{
"inline_data": {
"mime_type":"'"${MIME2_TYPE}"'",
"data": "'"$IMAGE2_BASE64"'"
}
}
]
}]
}' 2> /dev/null > response.json
cat response.json
echo
jq ".candidates[].content.parts[].text" response.json
किसी ऑब्जेक्ट का बाउंडिंग बॉक्स पाना
Gemini मॉडल को इमेज में मौजूद ऑब्जेक्ट की पहचान करने और उनके बॉउंडिंग बॉक्स के निर्देशांक देने के लिए ट्रेन किया जाता है. निर्देशांक, इमेज के डाइमेंशन के हिसाब से दिखाए जाते हैं. इन्हें [0, 1000] तक स्केल किया जाता है. आपको अपनी मूल इमेज के साइज़ के आधार पर, इन कोऑर्डिनेट का स्केल कम करना होगा.
Python
prompt = "Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000."
JavaScript
const prompt = "Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000.";
शुरू करें
prompt := []genai.Part{
genai.FileData{URI: sampleImage.URI},
genai.Text("Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000."),
}
REST
PROMPT="Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000."
ऑब्जेक्ट का पता लगाने और इमेज और वीडियो में जगह की जानकारी पाने के लिए, बाउंडिंग बॉक्स का इस्तेमाल किया जा सकता है. बॉउंडिंग बॉक्स की मदद से, ऑब्जेक्ट की सटीक पहचान करके और उन्हें अलग-अलग दिखाकर, कई तरह के ऐप्लिकेशन इस्तेमाल किए जा सकते हैं. साथ ही, अपने प्रोजेक्ट की बेहतर जानकारी भी हासिल की जा सकती है.
मुख्य फ़ायदे
- आसान: अपने ऐप्लिकेशन में ऑब्जेक्ट की पहचान करने की सुविधाओं को आसानी से इंटिग्रेट करें. भले ही, आपके पास कंप्यूटर विज़न की विशेषज्ञता न हो.
- पसंद के मुताबिक बनाया जा सकता है: कस्टम निर्देशों (उदाहरण के लिए, "मुझे इस इमेज में हरे रंग के सभी ऑब्जेक्ट के बॉउंडिंग बॉक्स देखने हैं") के आधार पर बॉउंडिंग बॉक्स बनाए जा सकते हैं. इसके लिए, आपको कस्टम मॉडल को ट्रेन करने की ज़रूरत नहीं है.
तकनीकी जानकारी
- इनपुट: आपका प्रॉम्प्ट और उससे जुड़ी इमेज या वीडियो फ़्रेम.
- आउटपुट:
[y_min, x_min, y_max, x_max]
फ़ॉर्मैट में बॉउंडिंग बॉक्स. सबसे ऊपर बाईं ओर ऑरिजिन होता है.x
औरy
ऐक्सिस, क्रमशः हॉरिज़ॉन्टल और वर्टिकल होते हैं. हर इमेज के लिए, कोऑर्डिनेट वैल्यू को 0 से 1,000 के बीच सामान्य किया जाता है. - विज़ुअलाइज़ेशन: AI Studio के उपयोगकर्ताओं को यूज़र इंटरफ़ेस में प्लॉट किए गए बॉर्डर बॉक्स दिखेंगे.
Python डेवलपर के लिए, 2D स्पेसियल समझने वाली नोटबुक या एक्सपेरिमेंट के तौर पर उपलब्ध 3D पॉइंट करने वाली नोटबुक आज़माएं.
निर्देशांकों को सामान्य करना
मॉडल, बॉउंडिंग बॉक्स के निर्देशांक को [y_min, x_min, y_max, x_max]
फ़ॉर्मैट में दिखाता है. इन सामान्य किए गए निर्देशांकों को अपनी मूल इमेज के पिक्सल निर्देशांक में बदलने के लिए, यह तरीका अपनाएं:
- हर आउटपुट कोऑर्डिनेट को 1,000 से भाग दें.
- x-कोऑर्डिनेट को ओरिजनल इमेज की चौड़ाई से गुणा करें.
- y-कोऑर्डिनेट को ओरिजनल इमेज की ऊंचाई से गुणा करें.
बॉउंडिंग बॉक्स के निर्देशांक जनरेट करने और इमेज पर उन्हें विज़ुअलाइज़ करने के बारे में ज़्यादा जानकारी वाले उदाहरणों को देखने के लिए, ऑब्जेक्ट डिटेक्शन कुकबुक का उदाहरण देखें.
इमेज का सेगमेंटेशन
Gemini 2.5 मॉडल से शुरू होकर, Gemini मॉडल को सिर्फ़ आइटम का पता लगाने के लिए ही नहीं, बल्कि उन्हें सेगमेंट करने और उनके कॉन्टूर का मास्क देने के लिए भी ट्रेन किया जाता है.
मॉडल, JSON की एक सूची का अनुमान लगाता है. इसमें हर आइटम, सेगमेंटेशन मास्क को दिखाता है.
हर आइटम में [y0, x0, y1, x1]
फ़ॉर्मैट में एक बाउंडिंग बॉक्स ("box_2d
") होता है. इसमें ऑब्जेक्ट की पहचान करने वाला लेबल ("label
") और बाउंडिंग बॉक्स के अंदर, 0 से 255 के बीच की वैल्यू वाला प्रोबैबिलिटी मैप होता है. यह मैप, base64 एन्कोड किए गए png फ़ॉर्मैट में होता है.
बॉर्डिंग बॉक्स के डाइमेंशन से मैच करने के लिए, मास्क का साइज़ बदलना होगा. इसके बाद, इसे आपके कॉन्फ़िडेंस थ्रेशोल्ड (मिडपॉइंट के लिए 127) पर बाइनरी में बदलना होगा.
Python
prompt = """
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
"""
JavaScript
const prompt = `
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
`;
शुरू करें
prompt := []genai.Part{
genai.FileData{URI: sampleImage.URI},
genai.Text(`
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
`),
}
REST
PROMPT='''
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
'''
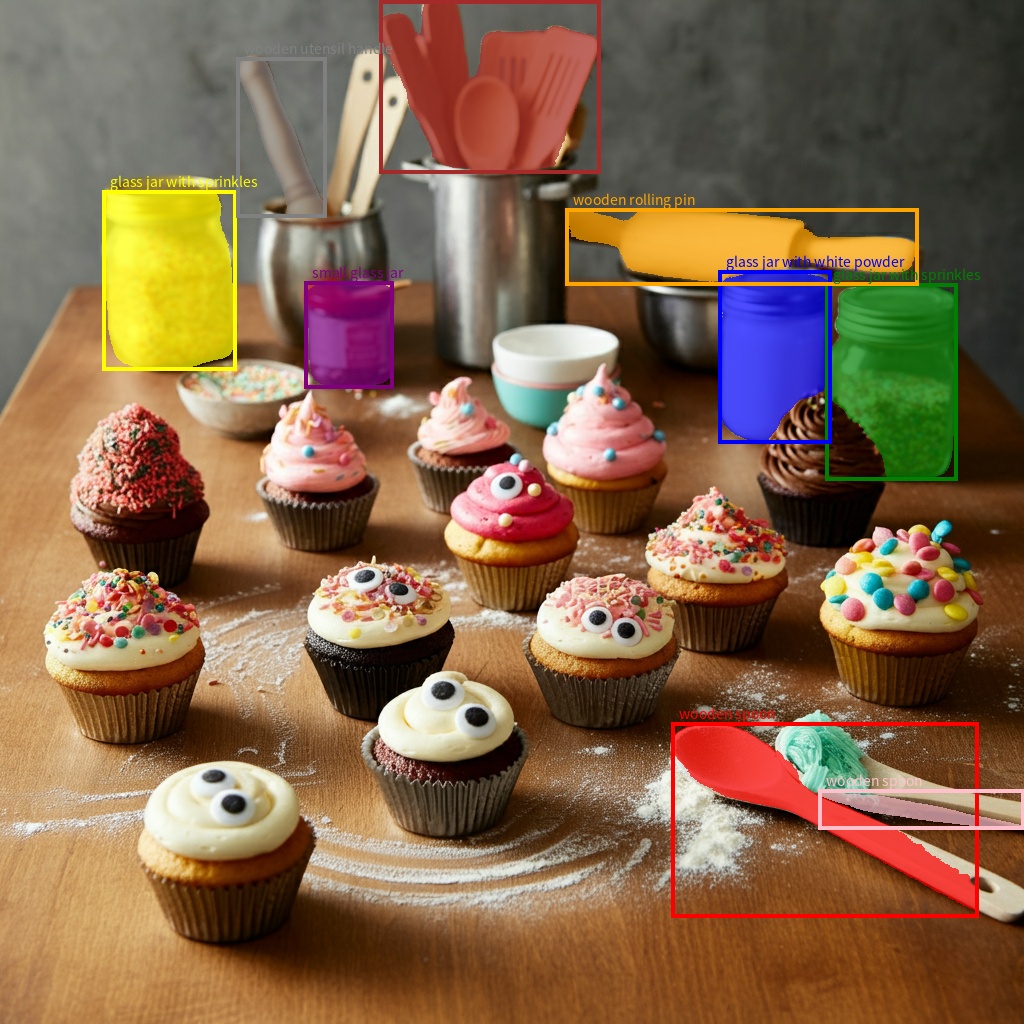
ज़्यादा जानकारी के लिए, कुकबुक गाइड में सेगमेंटेशन का उदाहरण देखें.
Google Images पर काम करने वाले फ़ॉर्मैट
Gemini, इमेज फ़ॉर्मैट के इन एमआईएमई टाइप के साथ काम करता है:
- PNG -
image/png
- JPEG -
image/jpeg
- WEBP -
image/webp
- HEIC -
image/heic
- HEIF -
image/heif
इमेज के बारे में तकनीकी जानकारी
- फ़ाइल की सीमा: Gemini 2.5 Pro, 2.0 Flash, 1.5 Pro, और 1.5 Flash में, हर अनुरोध के लिए ज़्यादा से ज़्यादा 3,600 इमेज फ़ाइलें इस्तेमाल की जा सकती हैं.
- टोकन का हिसाब लगाना:
- Gemini 1.5 Flash और Gemini 1.5 Pro: अगर दोनों डाइमेंशन <= 384 पिक्सल हैं, तो 258 टोकन. बड़ी इमेज को टाइल किया जाता है. टाइल का कम से कम साइज़ 256 पिक्सल और ज़्यादा से ज़्यादा 768 पिक्सल होता है. इमेज को 768x768 पिक्सल में बदला जाता है. हर टाइल की कीमत 258 टोकन होती है.
- Gemini 2.0 Flash: अगर दोनों डाइमेंशन 384 पिक्सल से कम हैं, तो 258 टोकन. बड़ी इमेज को 768x768 पिक्सल वाली टाइल में बांटा जाता है. हर टाइल की कीमत 258 टोकन होती है.
- सबसे सही तरीके:
- पक्का करें कि इमेज सही तरीके से घुमाई गई हों.
- साफ़ और धुंधली नहीं हुई इमेज का इस्तेमाल करें.
- टेक्स्ट वाली किसी एक इमेज का इस्तेमाल करते समय,
contents
कलेक्शन में टेक्स्ट प्रॉम्प्ट को इमेज के हिस्से के बाद रखें.
आगे क्या करना है
इस गाइड में, इमेज फ़ाइलें अपलोड करने और इमेज इनपुट से टेक्स्ट आउटपुट जनरेट करने का तरीका बताया गया है. ज़्यादा जानने के लिए, ये संसाधन देखें:
- सिस्टम के निर्देश: सिस्टम के निर्देशों की मदद से, अपनी ज़रूरतों और इस्तेमाल के उदाहरणों के आधार पर, मॉडल के व्यवहार को कंट्रोल किया जा सकता है.
- वीडियो को समझना: वीडियो इनपुट के साथ काम करने का तरीका जानें.
- Files API: Gemini के साथ इस्तेमाल करने के लिए, फ़ाइलें अपलोड करने और मैनेज करने के बारे में ज़्यादा जानें.
- फ़ाइल के लिए प्रॉम्प्ट करने की रणनीतियां: Gemini API, टेक्स्ट, इमेज, ऑडियो, और वीडियो डेटा के साथ प्रॉम्प्ट करने की सुविधा देता है. इसे मल्टीमॉडल प्रॉम्प्ट भी कहा जाता है.
- सुरक्षा से जुड़े दिशा-निर्देश: जनरेटिव एआई मॉडल कभी-कभी अनचाहे आउटपुट देते हैं. जैसे, गलत, पक्षपातपूर्ण या आपत्तिजनक आउटपुट. ऐसे आउटपुट से होने वाले नुकसान को कम करने के लिए, पोस्ट-प्रोसेसिंग और मानवीय आकलन करना ज़रूरी है.