يمكن لنماذج Gemini معالجة الصور، ما يتيح العديد من حالات الاستخدام المتقدّمة للمطوّرين التي كانت تتطلّب في السابق نماذج خاصة بنطاق معيّن. تشمل بعض ميزات الرؤية في Gemini ما يلي:
- إضافة تعليقات توضيحية إلى الصور والإجابة عن أسئلة حولها
- تحويل ملفات PDF إلى نص وتحليلها، بما في ذلك ما يصل إلى مليونَي رمز
- رصد الأجسام في صورة وعرض إحداثيات مربّع الحدود لها
- تقسيم العناصر ضمن صورة
تم تصميم Gemini ليكون متعدّد الوسائط من البداية، ونحن نواصل توسيع حدود ما هو ممكن. يوضّح هذا الدليل كيفية استخدام Gemini API لمحاولة توليد ردود نصية استنادًا إلى مدخلات الصور وتنفيذ المهام الشائعة لمحاولة فهم الصور.
قبل البدء
قبل استدعاء واجهة برمجة التطبيقات Gemini API، تأكَّد من تثبيت حزمة تطوير البرامج (SDK) المفضّلة لديك ، وضبط مفتاح واجهة برمجة التطبيقات Gemini API وجاهزيته للاستخدام.
إدخال صورة
يمكنك تقديم الصور كمدخلات إلى Gemini بالطُرق التالية:
- حمِّل ملف صورة باستخدام File API قبل إرسال
طلب إلى
generateContent
. استخدِم هذه الطريقة للملفات التي يزيد حجمها عن 20 ميغابايت أو عند الرغبة في إعادة استخدام الملف في طلبات متعددة. - أرسِل بيانات الصور المضمّنة مع الطلب إلى
generateContent
. استخدِم هذه الطريقة مع الملفات الأصغر حجمًا (حجم الطلب الإجمالي أقل من 20 ميغابايت) أو الصور التي يتم جلبها مباشرةً من عناوين URL.
تحميل ملف صورة
يمكنك استخدام Files API لتحميل ملف مصوّر. استخدِم دائمًا واجهة برمجة التطبيقات Files API عندما يكون حجم الطلب الإجمالي (بما في ذلك الملف، والطلب النصي، وتعليمات النظام، وما إلى ذلك) أكبر من 20 ميغابايت، أو إذا كنت تنوي استخدام الصورة نفسها في طلبات متعددة.
يحمِّل الرمز التالي ملف صورة، ثم يستخدم الملف في طلب موجَّه إلى
generateContent
.
Python
from google import genai
client = genai.Client(api_key="GOOGLE_API_KEY")
myfile = client.files.upload(file="path/to/sample.jpg")
response = client.models.generate_content(
model="gemini-2.0-flash",
contents=[myfile, "Caption this image."])
print(response.text)
JavaScript
import {
GoogleGenAI,
createUserContent,
createPartFromUri,
} from "@google/genai";
const ai = new GoogleGenAI({ apiKey: "GOOGLE_API_KEY" });
async function main() {
const myfile = await ai.files.upload({
file: "path/to/sample.jpg",
config: { mimeType: "image/jpeg" },
});
const response = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: createUserContent([
createPartFromUri(myfile.uri, myfile.mimeType),
"Caption this image.",
]),
});
console.log(response.text);
}
await main();
انتقال
file, err := client.UploadFileFromPath(ctx, "path/to/sample.jpg", nil)
if err != nil {
log.Fatal(err)
}
defer client.DeleteFile(ctx, file.Name)
model := client.GenerativeModel("gemini-2.0-flash")
resp, err := model.GenerateContent(ctx,
genai.FileData{URI: file.URI},
genai.Text("Caption this image."))
if err != nil {
log.Fatal(err)
}
printResponse(resp)
REST
IMAGE_PATH="path/to/sample.jpg"
MIME_TYPE=$(file -b --mime-type "${IMAGE_PATH}")
NUM_BYTES=$(wc -c < "${IMAGE_PATH}")
DISPLAY_NAME=IMAGE
tmp_header_file=upload-header.tmp
# Initial resumable request defining metadata.
# The upload url is in the response headers dump them to a file.
curl "https://generativelanguage.googleapis.com/upload/v1beta/files?key=${GOOGLE_API_KEY}" \
-D upload-header.tmp \
-H "X-Goog-Upload-Protocol: resumable" \
-H "X-Goog-Upload-Command: start" \
-H "X-Goog-Upload-Header-Content-Length: ${NUM_BYTES}" \
-H "X-Goog-Upload-Header-Content-Type: ${MIME_TYPE}" \
-H "Content-Type: application/json" \
-d "{'file': {'display_name': '${DISPLAY_NAME}'}}" 2> /dev/null
upload_url=$(grep -i "x-goog-upload-url: " "${tmp_header_file}" | cut -d" " -f2 | tr -d "\r")
rm "${tmp_header_file}"
# Upload the actual bytes.
curl "${upload_url}" \
-H "Content-Length: ${NUM_BYTES}" \
-H "X-Goog-Upload-Offset: 0" \
-H "X-Goog-Upload-Command: upload, finalize" \
--data-binary "@${IMAGE_PATH}" 2> /dev/null > file_info.json
file_uri=$(jq ".file.uri" file_info.json)
echo file_uri=$file_uri
# Now generate content using that file
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{"file_data":{"mime_type": "${MIME_TYPE}", "file_uri": '$file_uri'}},
{"text": "Caption this image."}]
}]
}' 2> /dev/null > response.json
cat response.json
echo
jq ".candidates[].content.parts[].text" response.json
للاطّلاع على مزيد من المعلومات عن العمل مع ملفات الوسائط، اطّلِع على Files API.
تمرير بيانات الصورة مضمّنة
بدلاً من تحميل ملف صورة، يمكنك تمرير بيانات الصورة المضمّنة في
الطلب إلى generateContent
. هذا الإجراء مناسب للصور الأصغر حجمًا
(حجم الطلب الإجمالي أقل من 20 ميغابايت) أو الصور التي يتم جلبها مباشرةً من عناوين URL.
يمكنك تقديم بيانات الصور كسلسة Base64 مشفّرة أو من خلال قراءة الملفات المحلية مباشرةً (حسب حزمة تطوير البرامج (SDK)).
ملف الصورة على الجهاز:
Python
from google.genai import types
with open('path/to/small-sample.jpg', 'rb') as f:
img_bytes = f.read()
response = client.models.generate_content(
model='gemini-2.0-flash',
contents=[
types.Part.from_bytes(
data=img_bytes,
mime_type='image/jpeg',
),
'Caption this image.'
]
)
print(response.text)
JavaScript
import { GoogleGenAI } from "@google/genai";
import * as fs from "node:fs";
const ai = new GoogleGenAI({ apiKey: "GOOGLE_API_KEY" });
const base64ImageFile = fs.readFileSync("path/to/small-sample.jpg", {
encoding: "base64",
});
const contents = [
{
inlineData: {
mimeType: "image/jpeg",
data: base64ImageFile,
},
},
{ text: "Caption this image." },
];
const response = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: contents,
});
console.log(response.text);
انتقال
model := client.GenerativeModel("gemini-2.0-flash")
bytes, err := os.ReadFile("path/to/small-sample.jpg")
if err != nil {
log.Fatal(err)
}
prompt := []genai.Part{
genai.Blob{MIMEType: "image/jpeg", Data: bytes},
genai.Text("Caption this image."),
}
resp, err := model.GenerateContent(ctx, prompt...)
if err != nil {
log.Fatal(err)
}
for _, c := range resp.Candidates {
if c.Content != nil {
fmt.Println(*c.Content)
}
}
REST
IMG_PATH=/path/to/your/image1.jpg
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{
"inline_data": {
"mime_type":"image/jpeg",
"data": "'\$(base64 \$B64FLAGS \$IMG_PATH)'"
}
},
{"text": "Caption this image."},
]
}]
}' 2> /dev/null
الصورة من عنوان URL:
Python
from google import genai
from google.genai import types
import requests
image_path = "https://goo.gle/instrument-img"
image = requests.get(image_path)
client = genai.Client(api_key="GOOGLE_API_KEY")
response = client.models.generate_content(
model="gemini-2.0-flash-exp",
contents=["What is this image?",
types.Part.from_bytes(data=image.content, mime_type="image/jpeg")])
print(response.text)
JavaScript
import { GoogleGenAI } from "@google/genai";
async function main() {
const ai = new GoogleGenAI({ apiKey: process.env.GOOGLE_API_KEY });
const imageUrl = "https://goo.gle/instrument-img";
const response = await fetch(imageUrl);
const imageArrayBuffer = await response.arrayBuffer();
const base64ImageData = Buffer.from(imageArrayBuffer).toString('base64');
const result = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: [
{
inlineData: {
mimeType: 'image/jpeg',
data: base64ImageData,
},
},
{ text: "Caption this image." }
],
});
console.log(result.text);
}
main();
انتقال
func main() {
ctx := context.Background()
client, err := genai.NewClient(ctx, option.WithAPIKey(os.Getenv("GOOGLE_API_KEY")))
if err != nil {
log.Fatal(err)
}
defer client.Close()
model := client.GenerativeModel("gemini-2.0-flash")
// Download the image.
imageResp, err := http.Get("https://goo.gle/instrument-img")
if err != nil {
panic(err)
}
defer imageResp.Body.Close()
imageBytes, err := io.ReadAll(imageResp.Body)
if err != nil {
panic(err)
}
// Create the request.
req := []genai.Part{
genai.ImageData("jpeg", imageBytes),
genai.Text("Caption this image."),
}
// Generate content.
resp, err := model.GenerateContent(ctx, req...)
if err != nil {
panic(err)
}
// Handle the response of generated text.
for _, c := range resp.Candidates {
if c.Content != nil {
fmt.Println(*c.Content)
}
}
}
REST
IMG_URL="https://goo.gle/instrument-img"
MIME_TYPE=$(curl -sIL "$IMG_URL" | grep -i '^content-type:' | awk -F ': ' '{print $2}' | sed 's/\r$//' | head -n 1)
if [[ -z "$MIME_TYPE" || ! "$MIME_TYPE" == image/* ]]; then
MIME_TYPE="image/jpeg"
fi
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{
"inline_data": {
"mime_type":"'"$MIME_TYPE"'",
"data": "'$(curl -sL "$IMG_URL" | base64 $B64FLAGS)'"
}
},
{"text": "Caption this image."}
]
}]
}' 2> /dev/null
في ما يلي بعض النقاط التي يجب أخذها في الاعتبار بشأن بيانات الصور المضمّنة:
- الحد الأقصى للحجم الإجمالي للطلب هو 20 ميغابايت، ويشمل ذلك الطلبات النصية وتعليمات النظام وجميع الملفات المقدَّمة مضمّنة. إذا كان حجم ملفك يؤدي إلى تجاوز إجمالي حجم الطلب 20 ميغابايت، استخدِم Files API لتحميل ملف صورة لاستخدامه في الطلب.
- إذا كنت تستخدِم عيّنة صورة عدّة مرّات، من الأفضل تحميل ملف صورة باستخدام File API.
طلب الموافقة باستخدام صور متعددة
يمكنك تقديم صور متعددة في طلب واحد عن طريق تضمين عناصر
Part
متعددة للصور في صفيف contents
. ويمكن أن تكون هذه البيانات مزيجًا من البيانات المضمّنة
(الملفات المحلية أو عناوين URL) ومراجع File API.
Python
from google import genai
from google.genai import types
client = genai.Client(api_key="GOOGLE_API_KEY")
# Upload the first image
image1_path = "path/to/image1.jpg"
uploaded_file = client.files.upload(file=image1_path)
# Prepare the second image as inline data
image2_path = "path/to/image2.png"
with open(image2_path, 'rb') as f:
img2_bytes = f.read()
# Create the prompt with text and multiple images
response = client.models.generate_content(
model="gemini-2.0-flash",
contents=[
"What is different between these two images?",
uploaded_file, # Use the uploaded file reference
types.Part.from_bytes(
data=img2_bytes,
mime_type='image/png'
)
]
)
print(response.text)
JavaScript
import {
GoogleGenAI,
createUserContent,
createPartFromUri,
} from "@google/genai";
import * as fs from "node:fs";
const ai = new GoogleGenAI({ apiKey: "GOOGLE_API_KEY" });
async function main() {
// Upload the first image
const image1_path = "path/to/image1.jpg";
const uploadedFile = await ai.files.upload({
file: image1_path,
config: { mimeType: "image/jpeg" },
});
// Prepare the second image as inline data
const image2_path = "path/to/image2.png";
const base64Image2File = fs.readFileSync(image2_path, {
encoding: "base64",
});
// Create the prompt with text and multiple images
const response = await ai.models.generateContent({
model: "gemini-2.0-flash",
contents: createUserContent([
"What is different between these two images?",
createPartFromUri(uploadedFile.uri, uploadedFile.mimeType),
{
inlineData: {
mimeType: "image/png",
data: base64Image2File,
},
},
]),
});
console.log(response.text);
}
await main();
انتقال
+ // Upload the first image
image1Path := "path/to/image1.jpg"
uploadedFile, err := client.UploadFileFromPath(ctx, image1Path, nil)
if err != nil {
log.Fatal(err)
}
defer client.DeleteFile(ctx, uploadedFile.Name)
// Prepare the second image as inline data
image2Path := "path/to/image2.png"
img2Bytes, err := os.ReadFile(image2Path)
if err != nil {
log.Fatal(err)
}
// Create the prompt with text and multiple images
model := client.GenerativeModel("gemini-2.0-flash")
prompt := []genai.Part{
genai.Text("What is different between these two images?"),
genai.FileData{URI: uploadedFile.URI},
genai.Blob{MIMEType: "image/png", Data: img2Bytes},
}
resp, err := model.GenerateContent(ctx, prompt...)
if err != nil {
log.Fatal(err)
}
printResponse(resp)
REST
# Upload the first image
IMAGE1_PATH="path/to/image1.jpg"
MIME1_TYPE=$(file -b --mime-type "${IMAGE1_PATH}")
NUM1_BYTES=$(wc -c < "${IMAGE1_PATH}")
DISPLAY_NAME1=IMAGE1
tmp_header_file1=upload-header1.tmp
curl "https://generativelanguage.googleapis.com/upload/v1beta/files?key=${GOOGLE_API_KEY}" \
-D upload-header1.tmp \
-H "X-Goog-Upload-Protocol: resumable" \
-H "X-Goog-Upload-Command: start" \
-H "X-Goog-Upload-Header-Content-Length: ${NUM1_BYTES}" \
-H "X-Goog-Upload-Header-Content-Type: ${MIME1_TYPE}" \
-H "Content-Type: application/json" \
-d "{'file': {'display_name': '${DISPLAY_NAME1}'}}" 2> /dev/null
upload_url1=$(grep -i "x-goog-upload-url: " "${tmp_header_file1}" | cut -d" " -f2 | tr -d "\r")
rm "${tmp_header_file1}"
curl "${upload_url1}" \
-H "Content-Length: ${NUM1_BYTES}" \
-H "X-Goog-Upload-Offset: 0" \
-H "X-Goog-Upload-Command: upload, finalize" \
--data-binary "@${IMAGE1_PATH}" 2> /dev/null > file_info1.json
file1_uri=$(jq ".file.uri" file_info1.json)
echo file1_uri=$file1_uri
# Prepare the second image (inline)
IMAGE2_PATH="path/to/image2.png"
MIME2_TYPE=$(file -b --mime-type "${IMAGE2_PATH}")
if [[ "$(base64 --version 2>&1)" = *"FreeBSD"* ]]; then
B64FLAGS="--input"
else
B64FLAGS="-w0"
fi
IMAGE2_BASE64=$(base64 $B64FLAGS $IMAGE2_PATH)
# Now generate content using both images
curl "https://generativelanguage.googleapis.com/v1beta/models/gemini-2.0-flash:generateContent?key=$GOOGLE_API_KEY" \
-H 'Content-Type: application/json' \
-X POST \
-d '{
"contents": [{
"parts":[
{"text": "What is different between these two images?"},
{"file_data":{"mime_type": "'"${MIME1_TYPE}"'", "file_uri": '$file1_uri'}},
{
"inline_data": {
"mime_type":"'"${MIME2_TYPE}"'",
"data": "'"$IMAGE2_BASE64"'"
}
}
]
}]
}' 2> /dev/null > response.json
cat response.json
echo
jq ".candidates[].content.parts[].text" response.json
الحصول على مربّع حدود لعنصر
تم تدريب نماذج Gemini على تحديد الأشياء في الصورة وتقديم إحداثيات مربّع الحدود. يتم عرض الإحداثيات بالنسبة إلى سمات الصورة، ويتم تحويلها إلى نطاق [0، 1000]. يجب إزالة مقياس هذه الإحداثيات استنادًا إلى حجم الصورة الأصلي.
Python
prompt = "Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000."
JavaScript
const prompt = "Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000.";
انتقال
prompt := []genai.Part{
genai.FileData{URI: sampleImage.URI},
genai.Text("Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000."),
}
REST
PROMPT="Detect the all of the prominent items in the image. The box_2d should be [ymin, xmin, ymax, xmax] normalized to 0-1000."
يمكنك استخدام المربّعات الحدودية لرصد الأجسام وتحديد موقعها الجغرافي في الصور والفيديوهات. من خلال تحديد الأجسام بدقة وتحديد حدودها باستخدام المربّعات المحدودِة، يمكنك الاستفادة من مجموعة كبيرة من التطبيقات وتحسين ذكاء مشاريعك.
المزايا الرئيسية
- بسيط: يمكنك دمج إمكانات رصد الأجسام في تطبيقاتك بسهولة، بغض النظر عن خبرتك في مجال الرؤية الحاسوبية.
- قابل للتخصيص: يمكنك إنشاء مربّعات حدودية استنادًا إلى تعليمات مخصّصة (مثل "أريد الاطّلاع على مربّعات حدودية لجميع الأجسام الخضراء في هذه الصورة")، بدون الحاجة إلى تدريب نموذج مخصّص.
التفاصيل الفنية
- الإدخال: الطلب والصور أو لقطات الفيديو المرتبطة به
- الإخراج: مربّعات الحدود بتنسيق
[y_min, x_min, y_max, x_max]
الزاوية العليا اليسرى هي نقطة الأصل. يمتد محوراx
وy
أفقيًا وعموديًا على التوالي. يتم توحيد قيم التنسيقات من 0 إلى 1000 لكل صورة. - العرض المرئي: سيرى مستخدمو AI Studio مربّعات حدودية مرسومة ضمن واجهة المستخدِم.
بالنسبة إلى مطوّري Python، جرِّب دفتر ملاحظات الفهم المكاني ثنائي الأبعاد أو دفتر ملاحظات التوجيه التجريبي الثلاثي الأبعاد.
توحيد الإحداثيات
يعرض النموذج إحداثيات المربّع المحدود بالتنسيق
[y_min, x_min, y_max, x_max]
. لتحويل هذه الإحداثيات المُعدَّلة
إلى إحداثيات البكسل لصورتك الأصلية، اتّبِع الخطوات التالية:
- اقسم كل إحداثي ناتج على 1000.
- اضرب إحداثيات x في عرض الصورة الأصلية.
- اضرب إحداثيات y في ارتفاع الصورة الأصلية.
لاستكشاف أمثلة أكثر تفصيلاً لإنشاء إحداثيات مربّع الحدود و عرضها على الصور، راجِع مثال كتاب الطبخ لميزة "اكتشاف الأجسام".
تقسيم الصور
بدءًا من نماذج Gemini 2.5، يتم تدريب نماذج Gemini ليس فقط على رصد العناصر، بل أيضًا تقسيمها وتوفير قناع لخطوطها الخارجية.
ويتنبّأ النموذج بقائمة JSON، حيث يمثّل كل عنصر قناع تقسيم.
يحتوي كل عنصر على مربّع حدود ("box_2d
") بتنسيق [y0, x0, y1, x1]
مع إحداثيات موحّدة تتراوح بين 0 و1000، وتصنيف ("label
") يحدِّد
الكائن، وأخيراً قناع التقسيم داخل مربّع الحدود، بتنسيق png مُشفَّر بترميز base64، وهو خريطة احتمالات تتضمّن قيمًا تتراوح بين 0 و255.
يجب إعادة ضبط حجم القناع ليتناسب مع أبعاد المربّع الحدودي، ثم
تحويله إلى ثنائي عند الحدّ الأدنى للثقة (127 للنقطة الوسطى).
Python
prompt = """
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
"""
JavaScript
const prompt = `
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
`;
انتقال
prompt := []genai.Part{
genai.FileData{URI: sampleImage.URI},
genai.Text(`
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
`),
}
REST
PROMPT='''
Give the segmentation masks for the wooden and glass items.
Output a JSON list of segmentation masks where each entry contains the 2D
bounding box in the key "box_2d", the segmentation mask in key "mask", and
the text label in the key "label". Use descriptive labels.
'''
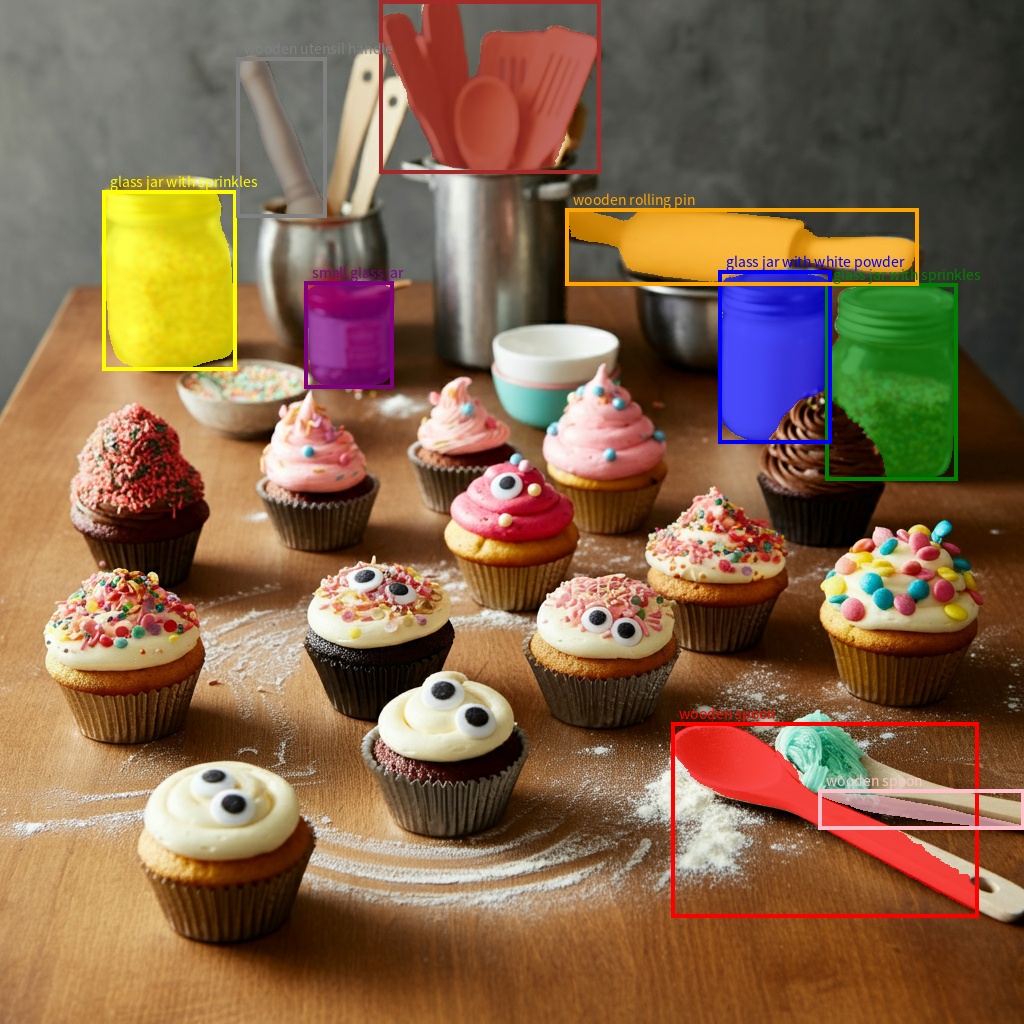
اطّلِع على مثال على التقسيم في دليل "كتاب الطبخ" للحصول على مثال أكثر تفصيلاً.
تنسيقات الصور المسموح بها
يتوافق Gemini مع أنواع MIME التالية لتنسيقات الصور:
- PNG -
image/png
- JPEG -
image/jpeg
- WEBP -
image/webp
- HEIC -
image/heic
- HEIF -
image/heif
التفاصيل الفنية حول الصور
- الحد الأقصى المسموح به للملفات: تتيح إصدارات Gemini 2.5 Pro و2.0 Flash و1.5 Pro و1.5 Flash تحميل ملفّات صور بعددٍ محدود يبلغ 3,600 ملفّ كحدّ أقصى لكلّ طلب.
- احتساب الرمز المميّز:
- Gemini 1.5 Flash وGemini 1.5 Pro: 258 رمزًا مميزًا إذا كانت كلتا السمتَين <= 384 بكسل يتم تقسيم الصور الأكبر حجمًا إلى مربّعات (الحد الأدنى للمربّع 256 بكسل والحد الأقصى 768 بكسل، ويتم تغيير حجمه إلى 768 × 768)، وتبلغ تكلفة كل مربّع 258 رمزًا ترويجيًا.
- Gemini 2.0 Flash: 258 رمزًا إذا كانت كلتا السمتَين <= 384 بكسل يتم تقسيم الصور الأكبر حجمًا إلى مربّعات بحجم 768 × 768 بكسل، وكلّ منها يكلف 258 رمزًا ترويجيًا.
- أفضل الممارسات:
- تأكَّد من أنّ الصور تم تدويرها بشكل صحيح.
- استخدِم صورًا واضحة وغير مموّهة.
- عند استخدام صورة واحدة تتضمّن نصًا، ضَع طلب النص بعد جزء الصورة في صفيف
contents
.
الخطوات التالية
يوضّح هذا الدليل كيفية تحميل ملفات الصور وإنشاء نصوص من مدخلات الصور. لمزيد من المعلومات، يُرجى الاطّلاع على المراجع التالية:
- تعليمات النظام: تتيح لك تعليمات النظام توجيه سلوك النموذج استنادًا إلى احتياجاتك وحالات الاستخدام المحدّدة.
- فهم الفيديو: تعرَّف على كيفية التعامل مع مدخلات الفيديو.
- Files API: اطّلِع على مزيد من المعلومات عن تحميل الملفات وإدارتها لاستخدامها مع Gemini.
- استراتيجيات طلب الملفات: تتيح واجهة برمجة التطبيقات Gemini API طلب البيانات النصية والمرئية والصوتية والفيديوية، والتي تُعرف أيضًا باسم طلبات البيانات المتعددة الوسائط.
- إرشادات السلامة: في بعض الأحيان، تُنتج نماذج الذكاء الاصطناعي التوليدي نتائج غير متوقّعة، مثل النتائج غير الدقيقة أو المتحيّزة أو المسيئة. إنّ المعالجة اللاحقة والتقييم البشري ضروريان لمحاولة الحد من خطر الضرر الناتج عن هذه النتائج.